RAL Classes
uvm_reg_block
The user-defined register block is derived from uvm_reg_block. It consists of all registers, maps, register files, and other register blocks if any. A block can have one or more address maps, each corresponding to a physical interface on the block. The register block at the highest hierarchical level provides the “Register Model” as an instance.
uvm_reg_block methods
Methods |
Description |
new |
Creates a new instance. |
configure |
Configuration for a specific instance. |
create_map |
Create an address map in the block |
set_default_map |
Defines the default address map |
lock_model |
Lock the model and build the address map |
get_block |
Get the sub-blocks |
get_map |
Get the address maps |
get_registers |
Get the registers |
get_fields |
Get the fields |
get_memories |
Get the memories |
reset |
Reset the mirror for the block |
update |
Update the mirrored values |
set_backdoor |
Set the user-defined backdoor for all registers in the block |
get_backdoor |
Get the user-defined backdoor for all registers in the block |
add_hdl_path |
Add the HDL path |
Note: Please refer to the UVM class reference manual to see all methods associated with the uvm_reg_block class
Example:
class RegModel_SFR extends uvm_reg_block;
// declaration for registers/ blocks/ register files/ memories/ address map
`uvm_object_utils(RegModel_SFR)
function new(string name = "RegModel_SFR");
super.new(name, ./*other arguments*/);
endfunction
virtual function void build();
// create registers/ blocks/ register files/ memories/ address map
endfunction
endclass
uvm_reg
The user-defined register class is derived from uvm_reg. It mimics the register of the design. To represent fields present in the register, RAL provides the uvm_reg_field class.
uvm_reg methods
Methods |
Description |
new |
Creates a new instance. |
configure |
Configuration for a specific instance. |
set_offset |
Modifies the register offset |
get_offset |
Returns the register offset |
get_address |
Returns the base external physical address of the register |
get_fields |
Returns the register fields |
set |
Sets the desired value of the register |
get |
Gets the desired value of the register |
reset |
Reset the desired/mirrored value of the register |
update |
Update the desired value to the register present in the DUT. |
read |
Read the current value from the register |
write |
Write the specified value in the register |
mirror |
Update the mirrored value for the register. |
set_backdoor |
Set the user-defined backdoor for all registers in the block |
get_backdoor |
Get the user-defined backdoor for all registers in the block |
add_hdl_path |
Add the HDL path |
Note: Please refer to the UVM class reference manual to see all methods associated with the uvm_reg class
Example:
class my_reg extends uvm_reg;
// declaration for register fields
`uvm_object_utils(my_reg)
function new(string name = "my_reg");
super.new(name, ./*other arguments*/);
endfunction
virtual function void build();
// create and configure register fields
endfunction
endclass
uvm_reg_field
It represents fields of the register. Each register can have one or more register fields that can have different access policies out of which RW, RO, W1C, W1S, etc are commonly used.
uvm_reg_field access policies
Access policies |
Description |
RO |
Read Only. It can simply read the register field value. Writing a field value has no effect |
RW |
Read Write: Field value can be written/modified and read the field value. |
W1C |
Write 1 to clear: 2. Writing 0 has no effect. 3. Reads field value |
W1S |
Write 1 to set: 2. Writing 0 has no effect. 3. Reads field value |
uvm_reg_field methods
Methods |
Description |
new |
Creates a new instance. |
configure |
Configuration for a specific instance. |
set_access |
Set the access policy of the field. |
get_access |
Get the access policy of the field. |
set |
Sets the desired value of the field |
get |
Gets the desired value of the field |
reset |
Reset the desired/mirrored value of the field |
read |
Read the current value from the field |
write |
Write the specified value in the field |
mirror |
Update the mirrored value for the field |
Note: Please refer to the UVM class reference manual to see all methods associated with the uvm_reg_field class
uvm_reg_file
The register file is derived from uvm_reg_file. The register file is nothing but a set of registers or register files.
uvm_reg_file methods
Methods |
Description |
new |
Creates a new instance. |
configure |
Configuration for a specific instance. |
get_regfile |
Get the parent register file |
add_hdl_path |
Add the HDL path |
Note: Please refer to the UVM class reference manual to see all methods associated with the uvm_reg_file class
uvm_mem
UVM RAL also supports memory implementation. As uvm_reg mimics registers of DUT. Similarly, uvm_mem mimics the memory of DUT.
uvm_mem methods
Methods |
Description |
new |
Creates a new instance. |
configure |
Configuration for a specific instance. |
set_offset |
Modifies the memory offset |
get_offset |
Returns the memory offset |
get_address |
Returns the base address of the memory location |
read |
Read the current value from the memory location |
write |
Write the specified value in the memory location |
set_backdoor |
Set the user-defined backdoor for the memory |
get_backdoor |
Get the user-defined backdoor for the memory |
add_hdl_path |
Add the HDL path |
Note: Please refer to the UVM class reference manual to see all methods associated with the uvm_mem class
uvm_reg_map
The user-defined uvm_reg_map is a base class used to access the model via a specific interface/bus.
uvm_reg_map methods
Methods |
Description |
new |
Creates a new instance. |
configure |
Configuration for a specific instance. |
add_reg |
Add a register |
add_mem |
Add a memory |
add_submap |
Add an address map |
reset |
Reset the mirror for all registers in this address map |
set_sequencer |
Set the sequencer and associated adapter for the map |
get_sequencer |
Get the sequencer and associated adapter for the map |
get_registers |
Get the registers |
get_reg_by_offset |
Get register mapped at offset |
get_mem_by_offset |
Get memory mapped at offset |
Note: Please refer to the UVM class reference manual to see all methods associated with the uvm_reg_map class
Set the sequencer and associated adapter for a single map
Example:
reg_model.axi_map.set_sequencer(.sequencer(agt.sequencer), .adapter(adapter) );
Where,
reg_model – register block
axi_map – address map
adapter – register adapter for converting register transactions to bus transaction (It will be discussed in RAL adapter section)
For multiple maps associated with different interfaces
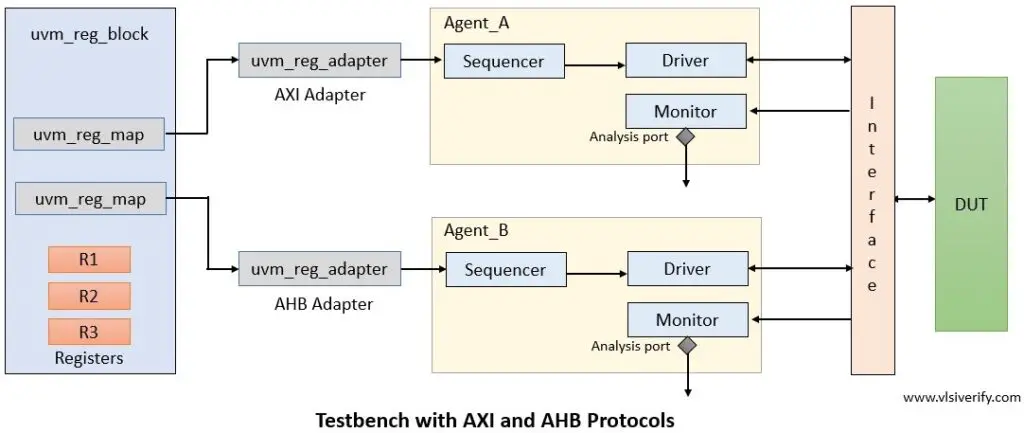
Example:
In the case of AXI and AHB interfaces, if the same RAL model has to be accessed, then a separate adapter class needs to be created. So, each register maps RAL model sequencer need to be set.
reg_model.axi_map.set_sequencer( .sequencer(axi_agt.sequencer), .adapter(axi_adapter) );
reg_model.ahb_map.set_sequencer( .sequencer(ahb_agt.sequencer), .adapter(ahb_adapter) );
Accessing a register of the different maps
reg_model.module_reg.control_sfr.write(.status(status), .value(wdata), .map(reg_model.axi_map));
reg_model.module_reg.control_sfr.write(.status(status), .value(wdata), .map(reg_model.ahb_map));
RAL Tutorials