Tutorials
Learn More
RAL Predictor
The UVM RAL predictor is a component that updates mirror values based on transactions on a physical interface for which UVM provides the ‘uvm_reg_predictor’ base class. The DUT registers can be updated either by RAL methods (like a read and write) or by running individual sequences with valid addresses and data on the target agent so that the driver communicates with DUT directly.
In front door access, UVM RAL provides three models for the predictor as
- Implicit (Auto) Prediction
- Explicit Prediction
- Passive Prediction
Auto Prediction
In auto prediction, front door access methods automatically call a predict() method for any transaction happening over a bus i.e. reading data from a register or writing data to the register at the end of the clock cycle.
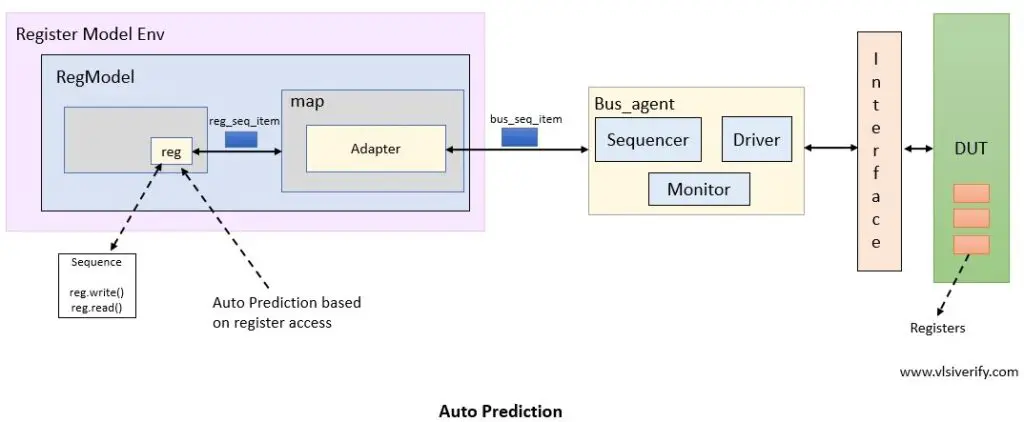
To enable auto prediction: Call set_auto_predict() method. The RAL model fails to update if method returns UVM_NOT_OK status
Advantage – Simple implementation
Disadvantage – It can not update the register model if register sequences are written to access DUT registers.
Explicit Prediction
It is the default mode of prediction that involves an explicit external predictor component that snoop for bus transactions and calls the predict() method to update its mirrored register value. Since it directly interacts with bus transactions, it requires a register adapter to convert bus transactions into a register transaction.
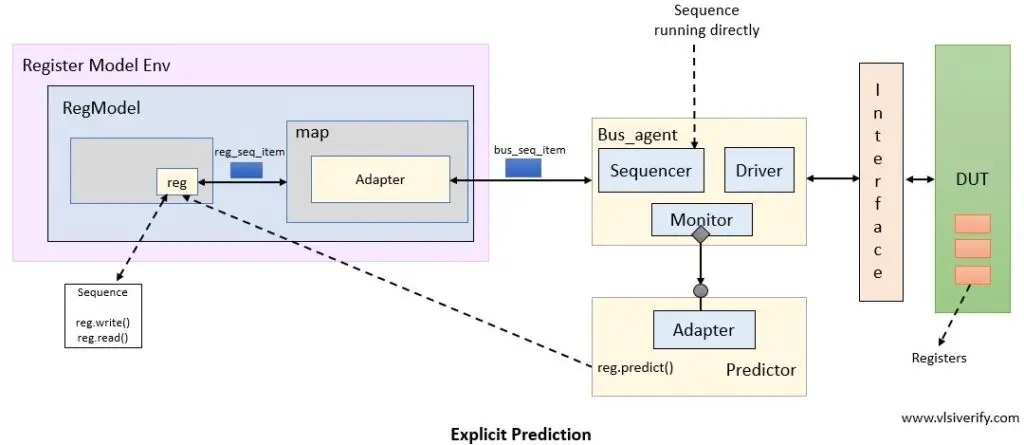
To enable explicit prediction: It is enabled by default.
//Create a predictor
uvm_reg_predictor #(axi_seq_item) axi_predictor;
axi_predictor = uvm_reg_predictor #(axi_seq_item) :: type_id:: create("axi_predictor", this);
//Configure
axi_predictor.map = reg_model.default_map; //Assigning map handle
axi_predictor.adapter = axi_reg_adapter; //Assigning adapter handle
//Connect
axi_mon.ap_mon.connect(axi_predictor.bus_imp); // Monitor analysis port and predictor analysis import connection
Advantage: This mode keeps the register model updated for transaction over the target bus interface
Disadvantage: Extra predictor component has to instantiate, configure and connect.
Plugin reg_predictor in testbench environment
class env extends uvm_env;
`uvm_component_utils(env)
agent agt;
reg_axi_adapter adapter;
uvm_reg_predictor #(axi_seq_item) axi_predictor;
RegModel_SFR reg_model; // Top level register block
function new(string name = "env", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
agt = agent::type_id::create("agt", this);
adapter = reg_axi_adapter::type_id::create("adapter");
axi_predictor = uvm_reg_predictor #(axi_seq_item) :: type_id:: create("axi_predictor", this);
reg_model = RegModel_SFR::type_id::create("reg_model");
...
endfunction
function void connect_phase(uvm_phase phase);
super.connect_phase(phase);
reg_model.default_map.set_sequencer( .sequencer(agt.seqr), .adapter(adapter) );
reg_model.default_map.set_base_addr('h0);
axi_predictor.map = reg_model.default_map; //Assigning map handle
axi_predictor.adapter = axi_reg_adapter; //Assigning adapter handle
axi_mon.ap_mon.connect(axi_predictor.bus_imp); // Monitor analysis port and predictor analysis import connection
endfunction
endclass
Passive Prediction
The passive prediction is similar to an explicit prediction that uses a predictor component except the front door register access methods can not be used.
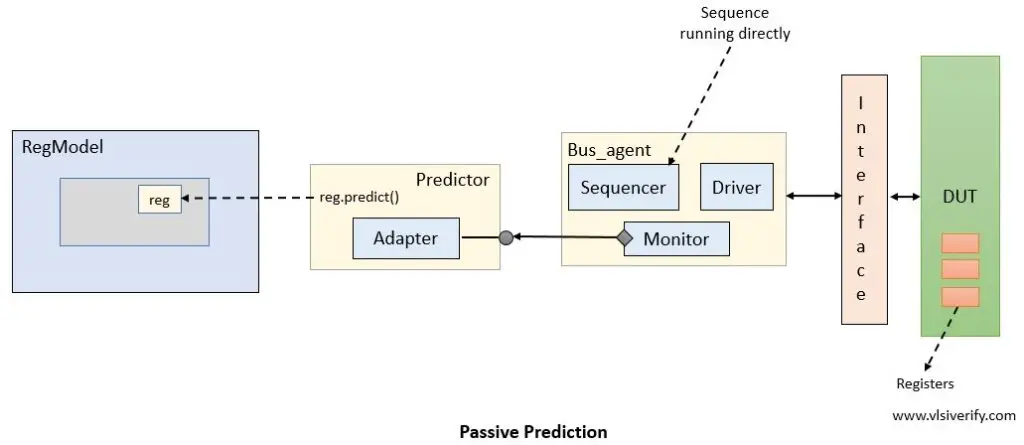
Advantage: This mode keeps the register model updated for transaction over the target bus interface
Disadvantage: front door register access methods can not be used. Only backdoor access is possible. Hence, it is rarely used.
RAL Tutorials