Tutorials
Learn More
Arbitration in Sequencer
The uvm_sequencer has a built-in mechanism to arbitrate within concurrently running sequences over the sequencer. Based on the arbitration algorithm, the sequencer sends sequence_item to the driver for the granted sequence.
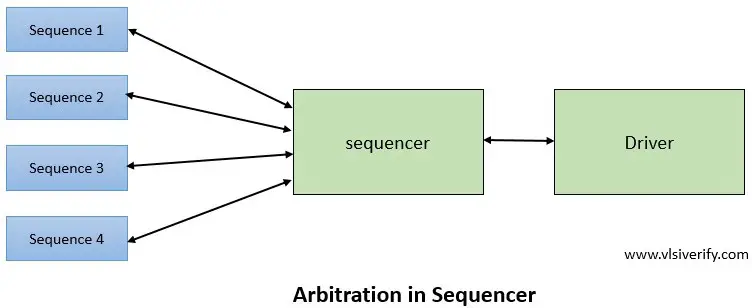
Each sequence sends sequence_items also do have its own id that differentiates from other sequences.
To set a particular arbitration mechanism, the set_arbitration function is used and it is defined in the uvm_sequencer_base class.
set_arbitration
It is used to specify the arbitration mode or mechanism for the sequencer.
function void set_arbitration( UVM_SEQ_ARB_TYPE val )
Any one of the below arbitration modes or algorithms can be selected.
For example:
<sequencer_name>.set_arbitration(UVM_SEQ_ARB_RANDOM);
Arbitration algorithms in uvm_sequencer
Algorithms |
Description |
UVM_SEQ_ARB_FIFO |
This is the default sequencer arbitration algorithm. It grants requests in FIFO order. |
UVM_SEQ_ARB_WEIGHTED |
Grant requests randomly by weight |
UVM_SEQ_ARB_RANDOM |
Grant requests randomly regardless of their priorities. |
UVM_SEQ_ARB_STRICT_FIFO |
Grant requests at the highest priority in FIFO order. |
UVM_SEQ_ARB_STRICT_RANDOM |
Grant requests randomly at the highest priority. |
UVM_SEQ_ARB_USER |
Grant requests based on user-defined function user_priority_arbitration written in the user-defined sequencer. |
get_arbitration
The get_arbitration function returns the current arbitration mode or algorithm set for the sequencer.
function UVM_SEQ_ARB_TYPE get_arbitration()
user_priority_arbitration
The sequencer will call the user_priority_arbitration function when arbitration mode is set to UVM_SEQ_ARB_USER using the set_arbitration function. The user-defined sequencer can override the user_priority_arbitration method to implement a customized arbitration policy.
virtual function integer user_priority_arbitration(integer avail_sequences[$])
Arbitration algorithms in uvm_sequencer examples
In the following examples, sequence_item is communicated to the driver using myseq. A base_test is written to have a common declaration and create an object (Ex: env class). A cfg_arb_mode task callback method is made available for the derived tests to update arbitration mode. Each flavor of the arbitration mode of the sequencer has a separate test and to execute on the EDA playground users have to pass proper test name as +UVM_TESTNAME = <test_name> in run options.
class myseq extends uvm_sequence #(seq_item);
seq_item req;
`uvm_object_utils(myseq)
function new (string name = "myseq");
super.new(name);
endfunction
task body();
`uvm_info(get_type_name(), "Inside body task", UVM_LOW);
req = seq_item::type_id::create("req");
wait_for_grant();
assert(req.randomize());
send_request(req);
wait_for_item_done();
`uvm_info(get_type_name(), "Completed body task", UVM_LOW);
endtask
endclass
The base_test without any priority to the sequencer
class base_test extends uvm_test;
env env_o;
myseq seq[5];
`uvm_component_utils(base_test)
function new(string name = "base_test", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
env_o = env::type_id::create("env_o", this);
endfunction
virtual task cfg_arb_mode;
endtask
task run_phase(uvm_phase phase);
string s_name;
super.run_phase(phase);
phase.raise_objection(this);
cfg_arb_mode();
`uvm_info(get_name, $sformatf("Arbitration mode = %s", env_o.agt.seqr.get_arbitration()), UVM_LOW);
foreach(seq[i]) begin
automatic int j = i;
fork
begin
s_name = $sformatf("seq[%0d]", j);
seq[j] = myseq::type_id::create(s_name);
seq[j].start(env_o.agt.seqr);
end
join_none
end
wait fork;
phase.drop_objection(this);
endtask
endclass
The base_test with priority to the sequencer
class base_test extends uvm_test;
env env_o;
myseq seq[5];
`uvm_component_utils(base_test)
function new(string name = "base_test", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
env_o = env::type_id::create("env_o", this);
endfunction
virtual task cfg_arb_mode;
endtask
task run_phase(uvm_phase phase);
string s_name;
super.run_phase(phase);
phase.raise_objection(this);
cfg_arb_mode();
`uvm_info(get_name, $sformatf("Arbitration mode = %s", env_o.agt.seqr.get_arbitration()), UVM_LOW);
foreach(seq[i]) begin
automatic int j = i;
fork
begin
s_name = $sformatf("seq[%0d]", j);
seq[j] = myseq::type_id::create(s_name);
seq[j].start(env_o.agt.seqr, .this_priority((j+1)*100)); // priority is mentioned as 100, 200, 300, 400, 500 for j = 0,1,2,3,4
end
join_none
end
wait fork;
phase.drop_objection(this);
endtask
endclass
Output for different arbitration modes
Test = seq_arb_fifo_test.sv and arbitration mode = UVM_SEQ_ARB_FIFO
Without sequencer priority
UVM_INFO @ 0: reporter [RNTST] Running test seq_arb_fifo_test...
UVM_INFO tests.sv(24) @ 0: uvm_test_top [uvm_test_top] Arbitration mode = UVM_SEQ_ARB_FIFO
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Inside body task
UVM_INFO driver.sv(17) @ 50: uvm_test_top.env_o.agt.drv [drv] addr = f92d and data = 1d95
UVM_INFO sequence.sv(16) @ 50: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 100: uvm_test_top.env_o.agt.drv [drv] addr = c6ed and data = c7af
UVM_INFO sequence.sv(16) @ 100: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 150: uvm_test_top.env_o.agt.drv [drv] addr = 252f and data = e872
UVM_INFO sequence.sv(16) @ 150: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 200: uvm_test_top.env_o.agt.drv [drv] addr = 40cc and data = 37af
UVM_INFO sequence.sv(16) @ 200: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 250: uvm_test_top.env_o.agt.drv [drv] addr = 76b4 and data = 6d4f
UVM_INFO sequence.sv(16) @ 250: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Completed body task
With sequencer priority
UVM_INFO @ 0: reporter [RNTST] Running test seq_arb_fifo_test...
UVM_INFO tests.sv(24) @ 0: uvm_test_top [uvm_test_top] Arbitration mode = UVM_SEQ_ARB_FIFO
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Inside body task
UVM_INFO driver.sv(17) @ 50: uvm_test_top.env_o.agt.drv [drv] addr = f92d and data = 1d95
UVM_INFO sequence.sv(16) @ 50: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 100: uvm_test_top.env_o.agt.drv [drv] addr = c6ed and data = c7af
UVM_INFO sequence.sv(16) @ 100: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 150: uvm_test_top.env_o.agt.drv [drv] addr = 252f and data = e872
UVM_INFO sequence.sv(16) @ 150: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 200: uvm_test_top.env_o.agt.drv [drv] addr = 40cc and data = 37af
UVM_INFO sequence.sv(16) @ 200: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 250: uvm_test_top.env_o.agt.drv [drv] addr = 76b4 and data = 6d4f
UVM_INFO sequence.sv(16) @ 250: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Completed body task
Test = seq_arb_weighted_test.sv and arbitration mode = UVM_SEQ_ARB_WEIGHTED
Without sequencer priority
UVM_INFO @ 0: reporter [RNTST] Running test seq_arb_weighted_test...
UVM_INFO tests.sv(24) @ 0: uvm_test_top [uvm_test_top] Arbitration mode = UVM_SEQ_ARB_WEIGHTED
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Inside body task
UVM_INFO driver.sv(17) @ 50: uvm_test_top.env_o.agt.drv [drv] addr = f92d and data = 1d95
UVM_INFO sequence.sv(16) @ 50: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 100: uvm_test_top.env_o.agt.drv [drv] addr = 252f and data = e872
UVM_INFO sequence.sv(16) @ 100: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 150: uvm_test_top.env_o.agt.drv [drv] addr = 40cc and data = 37af
UVM_INFO sequence.sv(16) @ 150: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 200: uvm_test_top.env_o.agt.drv [drv] addr = c6ed and data = c7af
UVM_INFO sequence.sv(16) @ 200: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 250: uvm_test_top.env_o.agt.drv [drv] addr = 76b4 and data = 6d4f
UVM_INFO sequence.sv(16) @ 250: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Completed body task
With sequencer priority
UVM_INFO @ 0: reporter [RNTST] Running test seq_arb_weighted_test...
UVM_INFO tests.sv(24) @ 0: uvm_test_top [uvm_test_top] Arbitration mode = UVM_SEQ_ARB_WEIGHTED
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Inside body task
UVM_INFO driver.sv(17) @ 50: uvm_test_top.env_o.agt.drv [drv] addr = f92d and data = 1d95
UVM_INFO sequence.sv(16) @ 50: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 100: uvm_test_top.env_o.agt.drv [drv] addr = 40cc and data = 37af
UVM_INFO sequence.sv(16) @ 100: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 150: uvm_test_top.env_o.agt.drv [drv] addr = c6ed and data = c7af
UVM_INFO sequence.sv(16) @ 150: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 200: uvm_test_top.env_o.agt.drv [drv] addr = 252f and data = e872
UVM_INFO sequence.sv(16) @ 200: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 250: uvm_test_top.env_o.agt.drv [drv] addr = 76b4 and data = 6d4f
UVM_INFO sequence.sv(16) @ 250: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Completed body task
Test = seq_arb_random_test.sv and arbitration mode = UVM_SEQ_ARB_RANDOM
Without sequencer priority
UVM_INFO @ 0: reporter [RNTST] Running test seq_arb_random_test...
UVM_INFO tests.sv(24) @ 0: uvm_test_top [uvm_test_top] Arbitration mode = UVM_SEQ_ARB_RANDOM
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Inside body task
UVM_INFO driver.sv(17) @ 50: uvm_test_top.env_o.agt.drv [drv] addr = 76b4 and data = 6d4f
UVM_INFO sequence.sv(16) @ 50: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 100: uvm_test_top.env_o.agt.drv [drv] addr = 252f and data = e872
UVM_INFO sequence.sv(16) @ 100: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 150: uvm_test_top.env_o.agt.drv [drv] addr = f92d and data = 1d95
UVM_INFO sequence.sv(16) @ 150: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 200: uvm_test_top.env_o.agt.drv [drv] addr = 40cc and data = 37af
UVM_INFO sequence.sv(16) @ 200: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 250: uvm_test_top.env_o.agt.drv [drv] addr = c6ed and data = c7af
UVM_INFO sequence.sv(16) @ 250: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Completed body task
With sequencer priority
UVM_INFO @ 0: reporter [RNTST] Running test seq_arb_random_test...
UVM_INFO tests.sv(24) @ 0: uvm_test_top [uvm_test_top] Arbitration mode = UVM_SEQ_ARB_RANDOM
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Inside body task
UVM_INFO driver.sv(17) @ 50: uvm_test_top.env_o.agt.drv [drv] addr = 76b4 and data = 6d4f
UVM_INFO sequence.sv(16) @ 50: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 100: uvm_test_top.env_o.agt.drv [drv] addr = 252f and data = e872
UVM_INFO sequence.sv(16) @ 100: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 150: uvm_test_top.env_o.agt.drv [drv] addr = f92d and data = 1d95
UVM_INFO sequence.sv(16) @ 150: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 200: uvm_test_top.env_o.agt.drv [drv] addr = 40cc and data = 37af
UVM_INFO sequence.sv(16) @ 200: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 250: uvm_test_top.env_o.agt.drv [drv] addr = c6ed and data = c7af
UVM_INFO sequence.sv(16) @ 250: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Completed body task
Test = seq_arb_strict_fifo_test.sv and arbitration mode = UVM_SEQ_ARB_STRICT_FIFO
Without sequencer priority
UVM_INFO @ 0: reporter [RNTST] Running test seq_arb_strict_fifo_test...
UVM_INFO tests.sv(24) @ 0: uvm_test_top [uvm_test_top] Arbitration mode = UVM_SEQ_ARB_STRICT_FIFO
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Inside body task
UVM_INFO driver.sv(17) @ 50: uvm_test_top.env_o.agt.drv [drv] addr = f92d and data = 1d95
UVM_INFO sequence.sv(16) @ 50: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 100: uvm_test_top.env_o.agt.drv [drv] addr = c6ed and data = c7af
UVM_INFO sequence.sv(16) @ 100: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 150: uvm_test_top.env_o.agt.drv [drv] addr = 252f and data = e872
UVM_INFO sequence.sv(16) @ 150: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 200: uvm_test_top.env_o.agt.drv [drv] addr = 40cc and data = 37af
UVM_INFO sequence.sv(16) @ 200: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 250: uvm_test_top.env_o.agt.drv [drv] addr = 76b4 and data = 6d4f
UVM_INFO sequence.sv(16) @ 250: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Completed body task
With sequencer priority
UVM_INFO @ 0: reporter [RNTST] Running test seq_arb_strict_fifo_test...
UVM_INFO tests.sv(24) @ 0: uvm_test_top [uvm_test_top] Arbitration mode = UVM_SEQ_ARB_STRICT_FIFO
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Inside body task
UVM_INFO driver.sv(17) @ 50: uvm_test_top.env_o.agt.drv [drv] addr = 76b4 and data = 6d4f
UVM_INFO sequence.sv(16) @ 50: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 100: uvm_test_top.env_o.agt.drv [drv] addr = 40cc and data = 37af
UVM_INFO sequence.sv(16) @ 100: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 150: uvm_test_top.env_o.agt.drv [drv] addr = 252f and data = e872
UVM_INFO sequence.sv(16) @ 150: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 200: uvm_test_top.env_o.agt.drv [drv] addr = c6ed and data = c7af
UVM_INFO sequence.sv(16) @ 200: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 250: uvm_test_top.env_o.agt.drv [drv] addr = f92d and data = 1d95
UVM_INFO sequence.sv(16) @ 250: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Completed body task
Test = seq_arb_strict_random_test.sv and arbitration mode = UVM_SEQ_ARB_STRICT_RANDOM
Without sequencer priority
UVM_INFO @ 0: reporter [RNTST] Running test seq_arb_strict_random_test...
UVM_INFO tests.sv(24) @ 0: uvm_test_top [uvm_test_top] Arbitration mode = UVM_SEQ_ARB_STRICT_RANDOM
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Inside body task
UVM_INFO driver.sv(17) @ 50: uvm_test_top.env_o.agt.drv [drv] addr = 76b4 and data = 6d4f
UVM_INFO sequence.sv(16) @ 50: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 100: uvm_test_top.env_o.agt.drv [drv] addr = 252f and data = e872
UVM_INFO sequence.sv(16) @ 100: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 150: uvm_test_top.env_o.agt.drv [drv] addr = f92d and data = 1d95
UVM_INFO sequence.sv(16) @ 150: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 200: uvm_test_top.env_o.agt.drv [drv] addr = 40cc and data = 37af
UVM_INFO sequence.sv(16) @ 200: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 250: uvm_test_top.env_o.agt.drv [drv] addr = c6ed and data = c7af
UVM_INFO sequence.sv(16) @ 250: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Completed body task
With sequencer priority
UVM_INFO @ 0: reporter [RNTST] Running test seq_arb_strict_random_test...
UVM_INFO tests.sv(24) @ 0: uvm_test_top [uvm_test_top] Arbitration mode = UVM_SEQ_ARB_STRICT_RANDOM
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Inside body task
UVM_INFO sequence.sv(10) @ 0: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Inside body task
UVM_INFO driver.sv(17) @ 50: uvm_test_top.env_o.agt.drv [drv] addr = 76b4 and data = 6d4f
UVM_INFO sequence.sv(16) @ 50: uvm_test_top.env_o.agt.seqr@@seq[4] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 100: uvm_test_top.env_o.agt.drv [drv] addr = 40cc and data = 37af
UVM_INFO sequence.sv(16) @ 100: uvm_test_top.env_o.agt.seqr@@seq[3] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 150: uvm_test_top.env_o.agt.drv [drv] addr = 252f and data = e872
UVM_INFO sequence.sv(16) @ 150: uvm_test_top.env_o.agt.seqr@@seq[2] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 200: uvm_test_top.env_o.agt.drv [drv] addr = c6ed and data = c7af
UVM_INFO sequence.sv(16) @ 200: uvm_test_top.env_o.agt.seqr@@seq[1] [myseq] Completed body task
UVM_INFO driver.sv(17) @ 250: uvm_test_top.env_o.agt.drv [drv] addr = f92d and data = 1d95
UVM_INFO sequence.sv(16) @ 250: uvm_test_top.env_o.agt.seqr@@seq[0] [myseq] Completed body task
The following points can be noted and observed in the above logs
- UVM_SEQ_ARB_FIFO and UVM_SEQ_ARB_RANDOM arbitration modes do not modify sequence execution even if priorities are specified.
- UVM_SEQ_ARB_STRICT_FIFO without any priority mentioned and UVM_SEQ_ARB_FIFO modes provide the same outcome.
- UVM_SEQ_ARB_STRICT_FIFO mode outcome changes based on priorities.
- UVM_SEQ_ARB_RANDOM and UVM_SEQ_ARB_STRICT_RANDOM provide the same outcome if no priorities are mentioned.
UVM Tutorials