Tutorials
Learn More
Passing interface handle down the hierarchy in UVM
The axi_inf interface handle is stored or set in the configuration database with field_name as axi_interface from env class. Down the hierarchy, it is retrieved or gets in the driver class using the same field_name.
set method call
class env extends uvm_env;
interface axi_if axi3_inf (clk , reset);
...
`uvm_component_utils(env)
function new(string name = "env", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
uvm_config_db #(virtual axi_if)::set(null, "*", "axi_interface", axi3_inf );
...
endfunction
...
...
endclass
get method call
class my_driver extends uvm_agent;
virtual interface axi_if axi3_vif;;
...
`uvm_component_utils(my_driver )
function new(string name = "my_driver", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
uvm_config_db #(virtual axi_if)::get(this, "*", "axi_interface", axi3_vif)
...
endfunction
...
...
endclass
Example with two agents
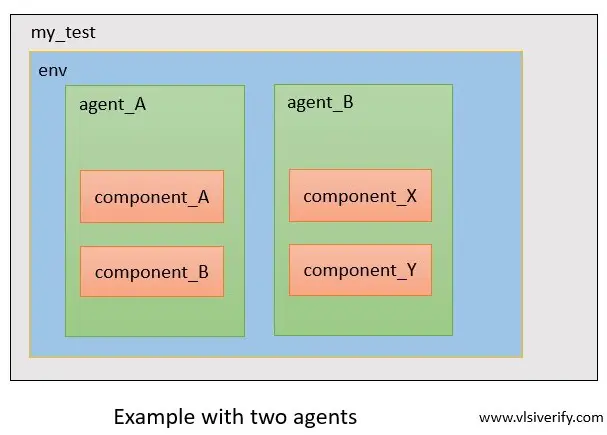
A set() call is used to store resources with field_name = value for hierarchy as uvm_test_top.env_o.agt_A.*
The get() is called in
- uvm_test_top.env_o – Error
- uvm_test_top.env_o.agt_A.comp_A and uvm_test_top.env_o.agt_A.comp_B – Pass
- uvm_test_top.env_o.agt_B.comp_X and uvm_test_top.env_o.agt_B.comp_Y – Error
agent_A.sv
class component_A extends uvm_component;
`uvm_component_utils(component_A)
int receive_value;
function new(string name = "component_A", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
if(!uvm_config_db #(int)::get(this, "*", "value", receive_value)) begin
`uvm_error(get_type_name(), "get failed for resource in this scope");
end
else begin
`uvm_info(get_full_name(), $sformatf("received value = %0d", receive_value), UVM_LOW);
end
endfunction
endclass
class component_B extends uvm_component;
int receive_value;
`uvm_component_utils(component_B)
function new(string name = "component_B", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
if(!uvm_config_db #(int)::get(this, "*", "value", receive_value)) begin
`uvm_error(get_type_name(), "get failed for resource in this scope");
end
else begin
`uvm_info(get_full_name(), $sformatf("received value = %0d", receive_value), UVM_LOW);
end
endfunction
endclass
class agent_A extends uvm_agent;
component_A comp_A;
component_B comp_B;
`uvm_component_utils(agent_A)
function new(string name = "component_A", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
comp_A = component_A ::type_id::create("comp_A", this);
comp_B = component_B ::type_id::create("comp_B", this);
endfunction
endclass
agent_B.sv
class component_X extends uvm_component;
`uvm_component_utils(component_X)
int receive_value;
function new(string name = "component_X", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
if(!uvm_config_db #(int)::get(this, "*", "value", receive_value)) begin
`uvm_error(get_type_name(), "get failed for resource in this scope");
end
else begin
`uvm_info(get_full_name(), $sformatf("received value = %0d", receive_value), UVM_LOW);
end
endfunction
endclass
class component_Y extends uvm_component;
int receive_value;
`uvm_component_utils(component_Y)
function new(string name = "component_Y", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
if(!uvm_config_db #(int)::get(this, "*", "value", receive_value)) begin
`uvm_error(get_type_name(), "get failed for resource in this scope");
end
else begin
`uvm_info(get_full_name(), $sformatf("received value = %0d", receive_value), UVM_LOW);
end
endfunction
endclass
class agent_B extends uvm_agent;
component_X comp_X;
component_Y comp_Y;
`uvm_component_utils(agent_B)
function new(string name = "agent_B", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
comp_X = component_X ::type_id::create("comp_X", this);
comp_Y = component_Y ::type_id::create("comp_Y", this);
endfunction
endclass
env.sv
class env extends uvm_env;
`uvm_component_utils(env)
agent_A agt_A;
agent_B agt_B;
int receive_value;
function new(string name = "env", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
agt_A = agent_A ::type_id::create("agt_A", this);
agt_B = agent_B ::type_id::create("agt_B", this);
if(!uvm_config_db #(int)::get(this, "*", "value", receive_value)) begin
`uvm_error(get_type_name(), "get failed for resource in this scope");
end
else begin
`uvm_info(get_full_name(), $sformatf("received value = %0d", receive_value), UVM_LOW);
end
endfunction
endclass
`include "uvm_macros.svh"
import uvm_pkg::*;
`include "agent_A.sv"
`include "agent_B.sv"
`include "env.sv"
class my_test extends uvm_test;
bit control;
`uvm_component_utils(my_test)
env env_o;
function new(string name = "my_test", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
env_o = env::type_id::create("env_o", this);
//uvm_config_db #(int)::set(null, "uvm_test_top.env_o.agt_A.*", "value", 100);
//or
uvm_config_db #(int)::set(this, "env_o.agt_A.*", "value", 100);
endfunction
function void end_of_elaboration_phase(uvm_phase phase);
super.end_of_elaboration_phase(phase);
uvm_top.print_topology();
endfunction
endclass
module tb_top;
initial begin
run_test("my_test");
end
endmodule
Output:
UVM_ERROR env.sv(17) @ 0: uvm_test_top.env_o [env] get failed for resource in this scope
UVM_INFO agent_A.sv(15) @ 0: uvm_test_top.env_o.agt_A.comp_A [uvm_test_top.env_o.agt_A.comp_A] received value = 100
UVM_INFO agent_A.sv(35) @ 0: uvm_test_top.env_o.agt_A.comp_B [uvm_test_top.env_o.agt_A.comp_B] received value = 100
UVM_ERROR agent_B.sv(12) @ 0: uvm_test_top.env_o.agt_B.comp_X [component_X] get failed for resource in this scope
UVM_ERROR agent_B.sv(32) @ 0: uvm_test_top.env_o.agt_B.comp_Y [component_Y] get failed for resource in this scope
UVM_FATAL @ 0: reporter [BUILDERR] stopping due to build errors
UVM Tutorials