UVM Command Line Processor (CLP)
With increasing complexity in the design and verification environment, the compilation time has also increased which also affects the verification timeline. Hence, there is a requirement to optimize it which can consider new configurations or parameters without forcing a recompilation. We have seen how a function or task behaves based on passing arguments. Similarly, UVM provides an interface to provide command-line arguments that provide flexibility to avoid testbench recompilation with the help of the ‘uvm_cmdline_processor’ class. It allows running a test with different configurations.
The uvm_cmdline_processor class provides setting various UVM variables from the command line such as components verbosity and configurations for integral types and strings.
uvm_cmdline_processor class hierarchy
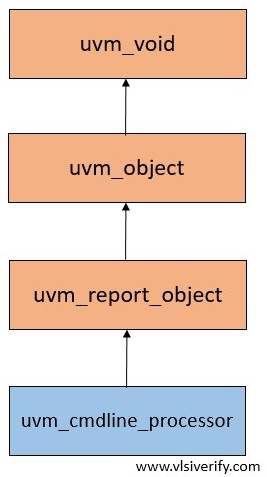
uvm_cmdline_processor Methods
Few methods of the command line processor and built-in command-line arguments are discussed here.
Type |
Methods |
Description |
function |
get_inst |
Returns a singleton instance of the UVM command line processor |
function |
get_args |
Returns a queue with all command-line arguments |
function |
get_plusargs |
Returns a queue with all plus arguments |
function |
get _uvmargs |
Returns a queue with all uvm arguments |
function |
get_args_value |
Finds the first argument which matches the ‘match’ argument and returns the suffix of the argument.’ |
`include "uvm_macros.svh"
import uvm_pkg::*;
class my_test extends uvm_test;
uvm_cmdline_processor clp;
bit [31:0] addr;
string clp_addr_str;
string clp_pattern_str;
string pattern;
`uvm_component_utils(my_test)
function new(string name = "my_test", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
clp = uvm_cmdline_processor::get_inst();
addr = clp.get_arg_value("+ADDR=", clp_addr_str) ? clp_addr_str.atohex(): 32'hF;
pattern = clp.get_arg_value("+PATTERN=", clp_pattern_str) ? clp_pattern_str: "Hello";
endfunction
function void end_of_elaboration_phase(uvm_phase phase);
super.end_of_elaboration_phase(phase);
`uvm_info(get_full_name(), $sformatf("addr = %0h, pattern = %s", addr, pattern), UVM_LOW)
endfunction
endclass
module tb_top;
initial begin
run_test("my_test");
end
endmodule
Please check “Run Options” while running the code.
Output:
UVM_INFO testbench.sv(25) @ 0: uvm_test_top [uvm_test_top] addr = aa, pattern = World
Built-in command line arguments in UVM
Command-line arguments |
Description |
+UVM_TESTNAME |
+UVM_TESTNAME = |
+UVM_VERBOSITY |
+UVM_VERBOSITY = |
+UVM_TIMEOUT |
+UVM_TIMEOUT= |
System functions for command line arguments in SystemVerilog
Similar to uvm_cmdline_processor class methods, System Verilog also provides some system functions.
System Functions |
Description |
$test$plusargs( |
Searches in the list of arguments for specified user strings. For a successful match, it returns a non-zero integer otherwise returns zero. |
$value$plusargs( |
Get the value for the specified user string in the variable. For a successful match for the given string, it returns a non-zero integer and store value in the variable otherwise returns zero. |
module cmd_line_args_ex;
bit [31:0] addr_1, addr_2;
string pattern;
initial begin
// +ADDR argument uses $test$plusargs and $value$plusargs
if($test$plusargs("ADDR="))
void'($value$plusargs("ADDR=%0h", addr_1));
else
addr_1 = 'hF;
if($test$plusargs("PATTERN="))
void'($value$plusargs("PATTERN=%s", pattern));
else
pattern = "Hello";
$display("address = %0h, pattern = %s", addr_1, pattern);
if($test$plusargs("ENABLE"))
$display("You have successfully received ENABLE as argument");
// +ADDR argument uses $value$plusargs only
if($value$plusargs("ADDR=%0h", addr_2))
$display("You have successfully received %0h value for ADDR argument", addr_2);
end
endmodule
Please check “Run Options” while running the code.
Output:
address = aa, pattern = World
You have successfully received ENABLE as argument
You have successfully received aa value for ADDR argument
UVM Tutorials