UVM Printer
The uvm_printer class provides flexibility to print uvm_objects in different formats. We have discussed the print() method using `uvm_field_* macro or write do_print() method if utils_begin/ end macros are not used.
The UVM printer provides four built-in printers.
- uvm_printer
- uvm_table_printer
- uvm_tree_printer
- uvm_line_printer
uvm_printer hierarchy
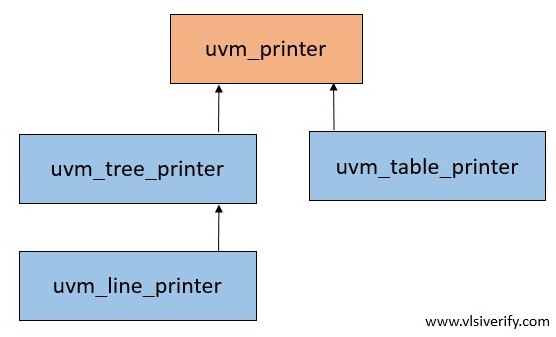
UVM Printer Classes
Printer classes |
Global instances |
Description |
uvm_printer |
uvm_printer uvm_default_printer = uvm_default_table_printer |
Provides base printer functionality |
uvm_table_printer |
uvm_table_printer uvm_default_table_printer = new() |
Prints the object in a tabular form |
uvm_tree_printer |
uvm_tree_printer uvm_default_tree_printer = new() |
Prints the object in a tree form |
uvm_line_printer |
uvm_line_printer uvm_default_line_printer = new() |
Prints the information in a single line |
UVM printer knobs
The uvm_printer_knobs class provides extra feasibility for printer settings for all printer types.
uvm_printer_knobs knobs = new
Some variables declared in the uvm_printer_knobs class.
size: Controls whether to print the field’s size.
depth: Indicates recursive depth while printing an object.
UVM printer Methods
Methods |
Description |
virtual function void print_field ( string name, uvm_bitstream_t value, int size, uvm_radix_enum radix = UVM_NORADIX, byte scope_separator = “.”, string type_name = “” ) |
Prints an integral fields upto 4096 bits Name: field name Value: field value Size: number of bits of the field Radix: radix to use for printing scope_separator: To find the leaf name of the field. |
virtual function void print_field_int ( string name, uvm_integral_t value, int size, uvm_radix_enum radix = UVM_NORADIX, byte scope_separator = “.”, string type_name = “” ) |
Same as print_field except size is upto 64 bits |
virtual function void print_object ( string name, uvm_object value, byte scope_separator = ” .” ) |
Prints an object and it is recursed depending on depth knob setting. Field definition remains the same as print_field method. |
virtual function void print_string ( string name, string value, byte scope_separator = “.” ) |
Prints a string field. |
virtual function void print_time ( string name, time value, byte scope_separator = “.” ) |
Prints a time value. Name: field name Value: field value to print. The print format is subject to the $timeformat system task. |
virtual function void print_real ( string name, real value, byte scope_separator = “.” ) |
Prints a real field. |
virtual function void print_generic ( string name, string type_name, int size, string value, byte scope_separator = “.” ) |
Prints a generic field for mentioned name, type, size and value. |
`include "uvm_macros.svh"
import uvm_pkg::*;
typedef enum{RED, GREEN, BLUE} color_type;
class temp_class extends uvm_object;
rand bit [7:0] tmp_addr;
rand bit [7:0] tmp_data;
function new(string name = "temp_class");
super.new(name);
endfunction
`uvm_object_utils_begin(temp_class)
`uvm_field_int(tmp_addr, UVM_ALL_ON)
`uvm_field_int(tmp_data, UVM_ALL_ON)
`uvm_object_utils_end
endclass
class my_object extends uvm_object;
rand int value;
string names;
rand color_type colors;
rand byte data[4];
rand bit [7:0] addr;
rand temp_class tmp;
`uvm_object_utils_begin(my_object)
`uvm_field_int(value, UVM_ALL_ON)
`uvm_field_string(names, UVM_ALL_ON)
`uvm_field_enum(color_type, colors, UVM_ALL_ON)
`uvm_field_sarray_int(data, UVM_ALL_ON)
`uvm_field_int(addr, UVM_ALL_ON)
`uvm_field_object(tmp, UVM_ALL_ON)
`uvm_object_utils_end
function new(string name = "my_object");
super.new(name);
tmp = new();
this.names = "UVM";
endfunction
endclass
class my_test extends uvm_test;
`uvm_component_utils(my_test)
my_object obj;
bit packed_data_bits[];
byte unsigned packed_data_bytes[];
int unsigned packed_data_ints[];
my_object unpack_obj;
function new(string name = "my_test", uvm_component parent = null);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
obj = my_object::type_id::create("obj", this);
assert(obj.randomize());
`uvm_info(get_full_name(), "obj print without any argument", UVM_LOW);
// Knob setting.
uvm_default_printer.knobs.indent = 5;
uvm_default_printer.knobs.hex_radix = "0x";
obj.print();
`uvm_info(get_full_name(), "obj print with uvm_default_table_printer argument", UVM_LOW);
obj.print(uvm_default_table_printer);
`uvm_info(get_full_name(), "obj print with uvm_default_tree_printer argument", UVM_LOW);
obj.print(uvm_default_tree_printer);
`uvm_info(get_full_name(), "obj print with uvm_default_line_printer argument", UVM_LOW);
obj.print(uvm_default_line_printer);
endfunction
endclass
module tb_top;
initial begin
run_test("my_test");
end
endmodule
Output:
UVM_INFO testbench.sv(61) @ 0: uvm_test_top [uvm_test_top] obj print without any argument
--------------------------------------------------
Name Type Size Value
--------------------------------------------------
obj my_object - @349
value integral 32 0x1f135537
names string 3 UVM
colors color_type 32 GREEN
data sa(integral) 4 -
[0] integral 8 0x9f
[1] integral 8 0x33
[2] integral 8 0x12
[3] integral 8 0x9c
addr integral 8 0x2f
tmp temp_class - @350
tmp_addr integral 8 0x39
tmp_data integral 8 0xbd
--------------------------------------------------
UVM_INFO testbench.sv(68) @ 0: uvm_test_top [uvm_test_top] obj print with uvm_default_table_printer argument
--------------------------------------------------
Name Type Size Value
--------------------------------------------------
obj my_object - @349
value integral 32 0x1f135537
names string 3 UVM
colors color_type 32 GREEN
data sa(integral) 4 -
[0] integral 8 0x9f
[1] integral 8 0x33
[2] integral 8 0x12
[3] integral 8 0x9c
addr integral 8 0x2f
tmp temp_class - @350
tmp_addr integral 8 0x39
tmp_data integral 8 0xbd
--------------------------------------------------
UVM_INFO testbench.sv(70) @ 0: uvm_test_top [uvm_test_top] obj print with uvm_default_tree_printer argument
obj: (my_object@349) {
value: 'h1f135537
names: UVM
colors: GREEN
data: {
[0]: 'h9f
[1]: 'h33
[2]: 'h12
[3]: 'h9c
}
addr: 'h2f
tmp: (temp_class@350) {
tmp_addr: 'h39
tmp_data: 'hbd
}
}
UVM_INFO testbench.sv(72) @ 0: uvm_test_top [uvm_test_top] obj print with uvm_default_line_printer argument
obj: (my_object@349) { value: 'h1f135537 names: UVM colors: GREEN data: { [0]: 'h9f [1]: 'h33 [2]: 'h12 [3]: 'h9c } addr: 'h2f tmp: (temp_class@350) { tmp_addr: 'h39 tmp_data: 'hbd } }
The print function defined in the uvm_object class
The print function in the uvm_object actually takes an argument as uvm_printer class type.
function void uvm_object::print(uvm_printer printer=null);
if (printer==null)
printer = uvm_default_printer;
if (printer == null)
`uvm_error("NULLPRINTER","uvm_default_printer is null")
$fwrite(printer.knobs.mcd,sprint(printer));
endfunction
UVM Tutorials