Tutorials
Learn More
UVM Queue
The uvm_queue class builds a dynamic queue that to be allotted on-demand basis and passed by reference.
uvm_queue class declaration:
class uvm_queue #( type T = int ) extends uvm_object
uvm_queue class hierarchy
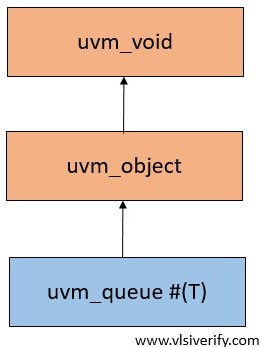
uvm_queue class Methods
Methods |
Description |
function new ( string name = “” ) |
Creates a new queue for the given name |
static function T get_global ( int index ) |
Returns the specified item instance from the global item queue. |
static function this_type get_global_queue () |
Returns the singleton global queue for the item of type T. |
virtual function int size () |
Returns size of the queue i.e. stored number of items in the queue. |
virtual function void insert ( int index, T item ) |
Inserts the item at the given index. |
virtual function void delete ( int index = -1 ) |
Removes the item from the given index. If an index is not provided, complete queue content will be deleted. |
virtual function T get ( int index ) |
Returns the item at the given index. |
virtual function void push_back( T item ) |
Inserts the given item at the back of the queue. |
virtual function void push_front( T item ) |
Inserts the given item at the front of the queue. |
virtual function T pop_back() |
Returns the last element in the queue or null if the queue is empty. |
virtual function T pop_front() |
Returns the first element in the queue or null if the queue is empty. |
UVM Queue Example
In the below example, componentA is used to add elements to the queue, and componentB is used to remove elements from the same queue.
componentA and componentB Code:
class componentA extends uvm_component;
`uvm_component_utils(componentA)
uvm_queue#(string) qA;
function new(string name = "componentA", uvm_component parent = null);
super.new(name, parent);
endfunction
task run_phase(uvm_phase phase);
super.run_phase(phase);
qA = uvm_queue#(string)::get_global_queue();
qA.push_front("Rock");
qA.push_back("Scissor");
qA.insert(1, "Paper");
endtask
endclass
class componentB extends uvm_component;
`uvm_component_utils(componentB)
uvm_queue#(string) qB;
string s_name;
function new(string name = "componentB", uvm_component parent = null);
super.new(name, parent);
endfunction
task run_phase(uvm_phase phase);
super.run_phase(phase);
s_name = uvm_queue#(string)::get_global(1);
`uvm_info(get_name(), $sformatf("get_global: item = %s", s_name), UVM_LOW);
qB = uvm_queue#(string)::get_global_queue();
s_name = qB.pop_front();
`uvm_info(get_name(), $sformatf("pop_front = %s", s_name), UVM_LOW);
`uvm_info(get_name(), $sformatf("Before delete: qB size = %0d", qB.size()), UVM_LOW);
qB.delete(1);
`uvm_info(get_name(), $sformatf("After delete: qB size = %0d", qB.size()), UVM_LOW);
s_name = qB.pop_back();
`uvm_info(get_name(), $sformatf("pop_back = %s", s_name), UVM_LOW);
endtask
endclass
class base_test extends uvm_test;
`uvm_component_utils(base_test)
componentA comp_a;
componentB comp_b;
function new(string name = "base_test",uvm_component parent=null);
super.new(name,parent);
endfunction : new
function void build_phase(uvm_phase phase);
super.build_phase(phase);
comp_a = componentA::type_id::create("comp_a", this);
comp_b = componentB::type_id::create("comp_b", this);
endfunction : build_phase
function void end_of_elaboration();
uvm_top.print_topology();
endfunction
endclass
module uvm_queue_example;
initial begin
run_test("base_test");
end
endmodule
Output:
UVM testbench topology:
-------------------------------------
Name Type Size Value
-------------------------------------
uvm_test_top base_test - @336
comp_a componentA - @349
comp_b componentB - @358
-------------------------------------
UVM_INFO components.sv(31) @ 0: uvm_test_top.comp_b [comp_b] get_global: item = Paper
UVM_INFO components.sv(36) @ 0: uvm_test_top.comp_b [comp_b] pop_front = Rock
UVM_INFO components.sv(38) @ 0: uvm_test_top.comp_b [comp_b] Before delete: qB size = 2
UVM_INFO components.sv(40) @ 0: uvm_test_top.comp_b [comp_b] After delete: qB size = 1
UVM_INFO components.sv(43) @ 0: uvm_test_top.comp_b [comp_b] pop_back = Paper
UVM Tutorials