UVM testbench Top
The testbench top is a static container that has an instantiation of DUT and interfaces. The interface instance connects with DUT signals in the testbench top. The clock is generated and initially reset is applied to the DUT. It is also passed to the interface handle. An interface is stored in the uvm_config_db using the set method and it can be retrieved down the hierarchy using the get method. UVM testbench top is also used to trigger a test using run_test() call.
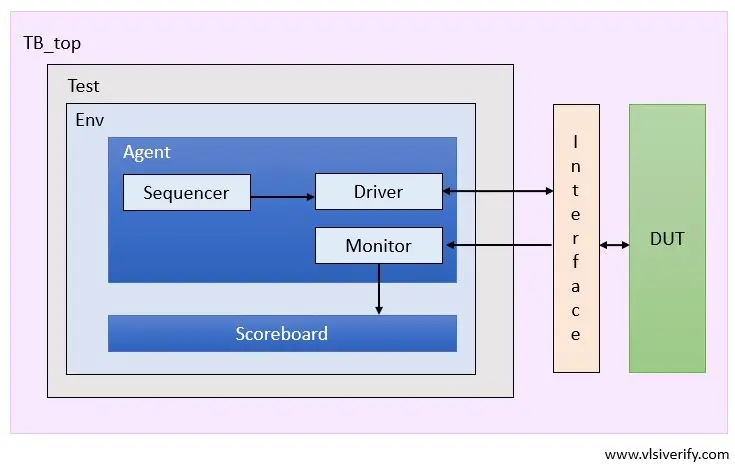
Example of UVM Testbench Top for Adder design
`include "uvm_macros.svh"
import uvm_pkg::*;
module tb_top;
bit clk;
bit reset;
always #5 clk = ~clk;
initial begin
clk = 0;
reset = 1;
#5;
reset = 0;
end
add_if vif(clk, reset);
// Instantiate design top
adder DUT(.clk(vif.clk),
.reset(vif.reset),
.in1(vif.ip1),
.in2(vif.ip2),
.out(vif.out)
);
initial begin
// set interface in config_db
uvm_config_db#(virtual add_if)::set(uvm_root::get(), "*", "vif", vif);
// Dump waves
$dumpfile("dump.vcd");
$dumpvars;
end
initial begin
run_test("base_test");
end
endmodule
UVM testbench |
Description |
UVM Test |
The test is at the top of the hierarchy that initiates the environment component construction. It is also responsible for the testbench configuration and stimulus generation process. |
UVM Environment |
An environment provides a well-mannered hierarchy and container for agents, scoreboards. |
UVM Agent |
An agent is a container that holds the driver, monitor, and sequencer. This is helpful to have a structured hierarchy based on the protocol or interface requirement |
UVM Sequence Item |
The transaction is a packet that is driven to the DUT or monitored by the monitor as a pin-level activity. |
UVM Driver |
The driver interacts with DUT. It receives randomized transactions or sequence items and drives them to the DUT as a pin-level activity. |
UVM Sequence |
The sequence is a container that holds data items (uvm_sequence_items) that are sent to or received from the driver via the sequencer. |
UVM Sequencer |
The sequencer is a mediator who establishes a connection between the sequence and the driver. Ultimately, it passes transactions or sequence items to the driver so that they can be driven to the DUT. |
UVM Monitor |
The monitor observes pin-level activity on the connected interface at the input and output of the design. This pin-level activity is converted into a transaction packet and sends to the scoreboard for checking purposes. |
UVM Scoreboard |
The scoreboard receives the transaction packet from the monitor and compares it with the reference model. The reference module is written based on design specification understanding and design behavior. |
UVM Testbench Top with multiple agents
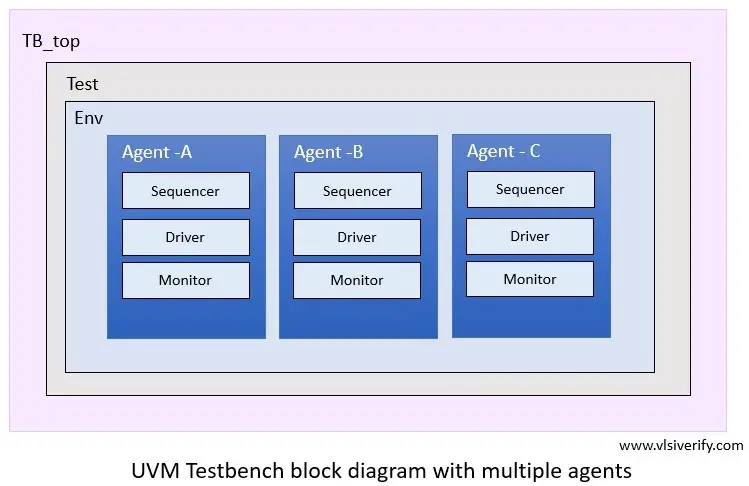
UVM Tutorials