Tutorials
Learn More
JK Flip Flop
The JK flip flop has two inputs ‘J’ and ‘K’. It behaves the same as SR flip flop except that it eliminates undefined output state (Q = x for S=1, R=1).
For J=1, K=1, output Q toggles from its previous output state.
Block Diagram
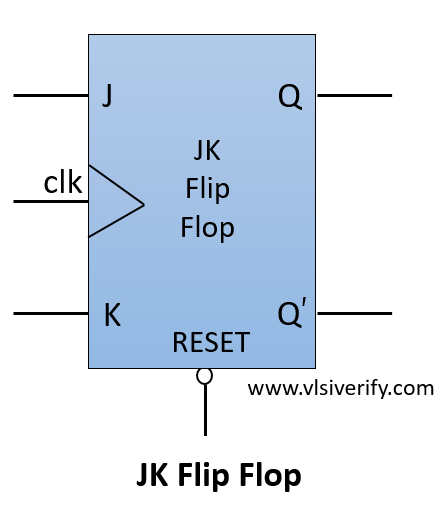
Truth Table
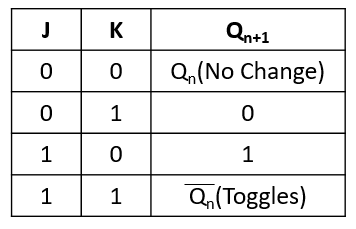
JK Flip Flop Verilog Code
module JK_flipflop (
input clk, rst_n,
input j,k,
output reg q,
output q_bar
);
// always@(posedge clk or negedge rst_n) // for asynchronous reset
always@(posedge clk) begin // for synchronous reset
if(!rst_n) q <= 0;
else begin
case({j,k})
2'b00: q <= q; // No change
2'b01: q <= 1'b0; // reset
2'b10: q <= 1'b1; // set
2'b11: q <= ~q; // Toggle
endcase
end
end
assign q_bar = ~q;
endmodule
Testbench Code
module tb;
reg clk, rst_n;
reg j, k;
wire q, q_bar;
JK_flipflop dff(clk, rst_n, j, k, q, q_bar);
always #2 clk = ~clk;
initial begin
clk = 0; rst_n = 0;
$display("Reset=%b --> q=%b, q_bar=%b", rst_n, q, q_bar);
#3 rst_n = 1;
$display("Reset=%b --> q=%b, q_bar=%b", rst_n, q, q_bar);
drive(2'b00);
drive(2'b01);
drive(2'b10);
drive(2'b11); // Toggles previous output
drive(2'b11); // Toggles previous output
#5;
$finish;
end
task drive(bit [1:0] ip);
@(posedge clk);
{j,k} = ip;
#1 $display("j=%b, k=%b --> q=%b, q_bar=%b",j, k, q, q_bar);
endtask
initial begin
$dumpfile("dump.vcd");
$dumpvars(1);
end
endmodule
Output:
Reset=0 --> q=x, q_bar=x
Reset=1 --> q=0, q_bar=1
j=0, k=0 --> q=0, q_bar=1
j=0, k=1 --> q=0, q_bar=1
j=1, k=0 --> q=1, q_bar=0
j=1, k=1 --> q=0, q_bar=1
j=1, k=1 --> q=1, q_bar=0
Verilog Codes