Tutorials
Learn More
Deep copy in SystemVerilog
The deep copy is the same as shallow copy except nested created objects are also copied by writing a custom method. Unlike shallow copy, full or deep copy performs a complete copy of an object.
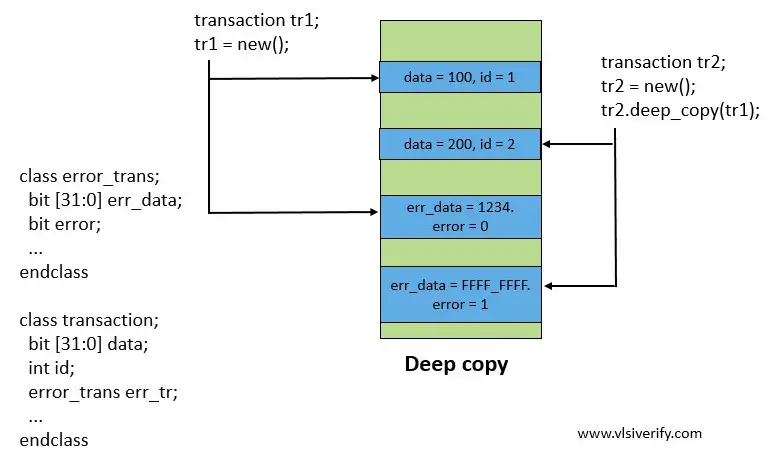
Deep copy Example
class error_trans;
bit [31:0] err_data;
bit error;
function new(bit [31:0] err_data, bit error);
this.err_data = err_data;
this.error = error;
endfunction
endclass
class transaction;
bit [31:0] data;
int id;
error_trans err_tr;
function new();
data = 100;
id = 1;
err_tr = new(32'hFFFF_FFFF, 1);
endfunction
function void display();
$display("transaction: data = %0d, id = %0d", data, id);
$display("error_trans: err_data = %0h, error = %0d\n", err_tr.err_data, err_tr.error);
endfunction
function deep_copy(transaction tr);
this.data = tr.data;
this.id = tr.id;
this.err_tr.err_data = tr.err_tr.err_data;
this.err_tr.error = tr.err_tr.error;
endfunction
endclass
module deep_copy_example;
transaction tr1, tr2;
initial begin
tr1 = new();
$display("Calling display method using tr1");
tr1.display();
tr2 = new();
tr2.deep_copy(tr1);
$display("After deep copy tr1 --> tr2");
$display("Calling display method using tr2");
tr2.display();
$display("--------------------------------");
tr1.data = 200;
tr1.id = 2;
tr1.err_tr.err_data = 32'h1234;
tr1.err_tr.error = 0;
$display("Calling display method using tr1");
tr1.display();
$display("Calling display method using tr2");
tr2.display();
end
endmodule
Output:
Calling display method using tr1
transaction: data = 100, id = 1
error_trans: err_data = ffffffff, error = 1
After deep copy tr1 --> tr2
Calling display method using tr2
transaction: data = 100, id = 1
error_trans: err_data = ffffffff, error = 1
--------------------------------
Calling display method using tr1
transaction: data = 200, id = 2
error_trans: err_data = 1234, error = 0
Calling display method using tr2
transaction: data = 100, id = 1
error_trans: err_data = ffffffff, error = 1
Highlights
Notice that
- The object tr2 is separately created.
- The deep_copy() extra method is written that does value assignments for an object which has to be copied (tr1 object). This ensures that tr1 class properties are copied to the newly created tr2 object.
System Verilog Tutorials