For loop in SystemVerilog
The for loop iterates till the mentioned condition is satisfied. The execution of for loop depends on –
- Initialization
- Condition
- Update
Syntax:
for (<initialization>; <condition>; <update>) begin
...
end
- Initialization: An initial value of the variable is set. It is executed only once.
- Condition: A condition or expression is evaluated. If it is evaluated to be the true body of for loop (statements inside begin and end) are executed else, the loop terminates.
- Update: After execution of for loop body, the variable value is updated
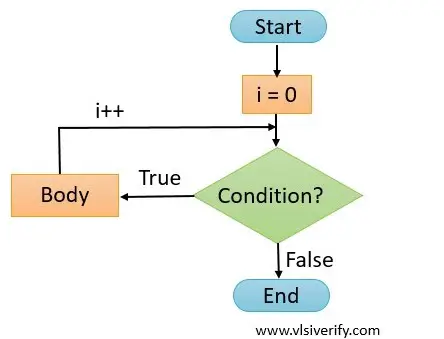
for loop flow chart
for loop Examples
A Basic for loop Example
module for_example;
int array[10];
initial begin
// Update array
for (int i = 0; i < $size(array); i++) begin
array[i] = i*i;
end
// Display array elements
for (int i = 0; i < $size(array); i++) begin
$display("array[%0d] = %0d", i, array[i]);
end
end
endmodule
Output:
array[0] = 0
array[1] = 1
array[2] = 4
array[3] = 9
array[4] = 16
array[5] = 25
array[6] = 36
array[7] = 49
array[8] = 64
array[9] = 81
Multiple variables in for loop
It is possible to have multiple variable initializations, conditions, and update values.
module for_example;
int array[10];
initial begin
// Update array
for (int i = 0, cnt = 0; i < $size(array); i++, cnt++) begin
array[i] = i*i;
$display("cnt = %0d", cnt);
end
// Display array elements
for (int i = 0; i < $size(array); i++) begin
$display("array[%0d] = %0d", i, array[i]);
end
end
endmodule
Output:
cnt = 0
cnt = 1
cnt = 2
cnt = 3
cnt = 4
cnt = 5
cnt = 6
cnt = 7
cnt = 8
cnt = 9
array[0] = 0
array[1] = 1
array[2] = 4
array[3] = 9
array[4] = 16
array[5] = 25
array[6] = 36
array[7] = 49
array[8] = 64
array[9] = 81
for loop behaves as a while loop
A for loop behaves as a while loop if initializations, conditions, and update values are not written.
System Verilog Tutorials